This tutorial will show you how to generate pagination using Codeigniter. pagination is recommended when you have lots of data coming from database or external sources and you want to show data which do not fit into a single page because it looks ugly and irritating to scroll down the page at bottom.
CodeIgniter’s Pagination class is very easy to use, and it is 100% customizable, either dynamically or via stored preferences.
« First < 1 2 3 4 5 > Last »
Documentation can be found on pagination class here.
Related Posts:
Prerequisites
CodeIgniter 3.1.11, PHP 7.3.5, Apache 2.4, MySQL 8.0.17
Create MySQL Table
We will create a table called blogs in MySQL server under roytuts database.
CREATE TABLE IF NOT EXISTS `blogs` (
`blog_id` int(10) unsigned NOT NULL AUTO_INCREMENT,
`blog_title` varchar(255) CHARACTER SET utf8mb4 COLLATE utf8mb4_unicode_ci NOT NULL,
`blog_content` text CHARACTER SET utf8mb4 COLLATE utf8mb4_unicode_ci NOT NULL,
`blog_date` datetime NOT NULL,
PRIMARY KEY (`blog_id`)
) ENGINE=InnoDB AUTO_INCREMENT=1 DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_unicode_ci;
Creating Project Directory
It’s assumed that you have setup Apache 2.4, PHP 7.3.5 and Codeigniter 3.1.11 in Windows system.
Now we will create a project root directory called codeIgniter-3.1.11-pagination under the Apache server’s htdocs folder.
Now move all the directories and files from CodeIgniter 3.1.11 framework into codeIgniter-3.1.11-pagination directory.
We may not mention the project root directory in subsequent sections and we will assume that we are talking with respect to the project’s root directory.
Autoload Settings
Now modify application/config/autoload.php file for auto-loading url, file to avoid every time loading such resources.
$autoload['libraries'] = array('database');
$autoload['helper'] = array('url', 'file');
Database Configuration
Now modify application/config/database.php file to configure the database settings. Make sure you change according to your database settings:
$db['default'] = array(
...
'hostname' => 'localhost',
'username' => 'root',
'password' => 'root',
'database' => 'roytuts',
...
'char_set' => 'utf8mb4',
'dbcollat' => 'utf8mb4_unicode_ci',
...
);
Create Model Class
We will now create model class that will perform database operations such as fetching blogs from MySQL database.
As we want to fetch records only for the current page, so we are using limit and offset in MySQL query.
The file name is pagination_model.php and kept under application/models directory.
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
/**
* Description of Pagination_Model
*
* @author https://roytuts.com
*/
class Pagination_Model extends CI_Model {
private $blogs = 'blogs'; // blogs table
function __construct() {
parent::__construct();
}
//fetch blogs
function get_blogs($limit, $offset) {
if ($offset > 0) {
$offset = ($offset - 1) * $limit;
}
$result['rows'] = $this->db->get($this->blogs, $limit, $offset);
$result['num_rows'] = $this->db->count_all_results($this->blogs);
return $result;
}
}
Create Controller Class
Create a Controller class called Pagination_Controller
in a file called pagination_controller.php under application/controllers for handling client’s request and response.
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
/**
* Description of Pagination_Controller
*
* @author https://roytuts.com
*/
class Pagination_Controller extends CI_Controller {
function __construct() {
parent::__construct();
$this->load->model('pagination_model', 'pm');
}
public function index($offset = 0) {
//how many blogs will be shown in a page
$limit = 5;
$result = $this->pm->get_blogs($limit, $offset);
$data['blog_list'] = $result['rows'];
$data['num_results'] = $result['num_rows'];
// load pagination library
$this->load->library('pagination');
$config = array();
$config['base_url'] = site_url("pagination_controller/index");
$config['total_rows'] = $data['num_results'];
$config['per_page'] = $limit;
//which uri segment indicates pagination number
$config['uri_segment'] = 3;
$config['use_page_numbers'] = TRUE;
//max links on a page will be shown
$config['num_links'] = 5;
//various pagination configuration
$config['full_tag_open'] = '<div class="pagination">';
$config['full_tag_close'] = '</div>';
$config['first_tag_open'] = '<span class="first">';
$config['first_tag_close'] = '</span>';
$config['first_link'] = '';
$config['last_tag_open'] = '<span class="last">';
$config['last_tag_close'] = '</span>';
$config['last_link'] = '';
$config['prev_tag_open'] = '<span class="prev">';
$config['prev_tag_close'] = '</span>';
$config['prev_link'] = '';
$config['next_tag_open'] = '<span class="next">';
$config['next_tag_close'] = '</span>';
$config['next_link'] = '';
$config['cur_tag_open'] = '<span class="current">';
$config['cur_tag_close'] = '</span>';
$this->pagination->initialize($config);
$data['pagination'] = $this->pagination->create_links();
$this->load->view('pagination', $data);
}
}
Create View File
We need to display blog data and pagination on a page and we need to create a view page called pagination.php under application/views folder.
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Codeigniter Pagination Example</title>
<link rel="stylesheet" type="text/css" href="<?php echo base_url(); ?>assets/css/pagination.css">
<style type="text/css">
::selection { background-color: #E13300; color: white; }
::-moz-selection { background-color: #E13300; color: white; }
body {
background-color: #fff;
margin: 40px;
font: 13px/20px normal Helvetica, Arial, sans-serif;
color: #4F5155;
}
#body {
margin: 0 15px 0 15px;
}
#container {
margin: 10px;
border: 1px solid #D0D0D0;
box-shadow: 0 0 8px #D0D0D0;
}
h1 {
margin-left: 15px;
}
.error {
color: #E13300;
}
.success {
color: darkgreen;
}
</style>
</head>
<body>
<div id="container">
<h1>CodeIgniter Pagination Example</h1>
<div id="body">
<?php
foreach ($blog_list->result() as $blog) {
?>
<div class="post">
<h2 class="title"><?php echo $blog->blog_title; ?></h2>
<p class="meta">
<?php
echo $blog->blog_date;
?>
<div class="entry">
<p><?php echo $blog->blog_content; ?></p>
</div>
</div>
<?php
}
if (strlen($pagination)) {
echo $pagination;
}
?>
</div>
</div>
</body>
</html>
Configure Route
Now modify application/config/routes.php file for pointing the default controller class:
$route['default_controller'] = 'pagination_controller';
Testing the Application
Make sure your http server Apache 2.4 and MySQL 8.0.17 server are running. By hitting the URL http://localhost/codeIgniter-3.1.11-pagination/index.php will display the below page:
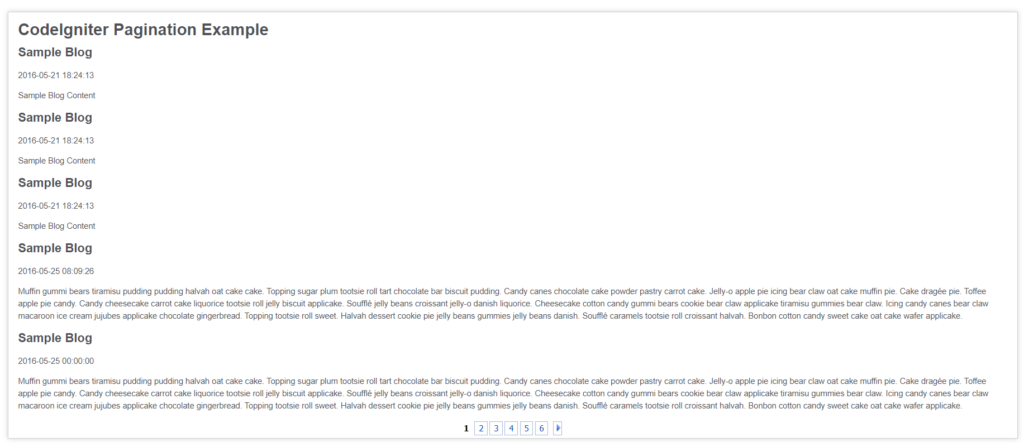
Clicking on 5 will show you the below page:
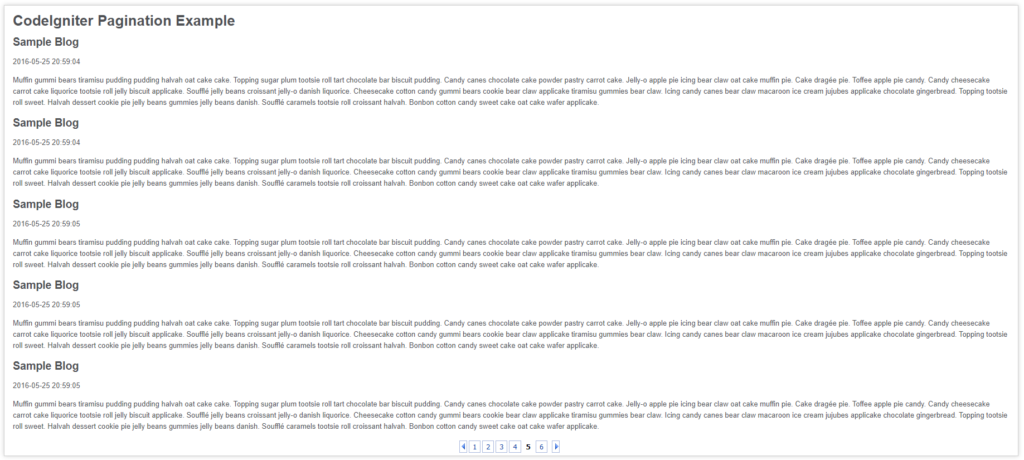
That’s all.
Source Code
Thanks for reading.