In this tutorial I will show you how to create a word document using apache poi or write to a word document using apache poi API. Microsoft word document is a great tool to document your stuff. This example is tested on both 3.15 and 4.1.1 versions of Apache POI library. I am also going to show you how to use build tools maven and gradle for the application.
We will create here a Java application to create word document using apache poi library. Using apache poi library is very easy for any kind of activities in word document.
Prerequisites
Eclipse 4.12, JDK 1.8 or 12, Maven 3.6.1, Gradle 5.6, Apache POI 3.15 to 4.1.1
Create Project
You basically need to create a maven or gradle project in eclipse. The name of the project is word-apache-poi.
Once the project is created and eclipse finishes build then you need to modify the pom.xml
file as shown below.
Here we will add apache poi API as a dependency for working with microsoft word document or even you can work with open source word document.
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.roytuts</groupId>
<artifactId>word-apache-poi</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<java.version>1.8</java.version>
<poi.version>3.15</poi.version>
</properties>
<dependencies>
<!-- apache poi for xlsx, docx etc reading/writing -->
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>${poi.version}</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<configuration>
<source>${java.version}</source>
<target>${java.version}</target>
</configuration>
</plugin>
</plugins>
</build>
</project>
If you want to use gradle build script to create your project then use below build script to setup the project. Here also we have used the same apache poi version as you have seen in the above maven based pom.xml file.
plugins {
id 'java-library'
}
sourceCompatibility = 12 or 1.8
targetCompatibility = 12 or 1.8
repositories {
jcenter()
}
dependencies {
implementation 'org.apache.poi:poi-ooxml:4.1.1 or 3.15'
}
Create Word File
Create below class to create WordDocx.docx
file.
The below source code creates a word document and writes sample texts to different paragraphs in different formats.
The first paragraph writes texts with justify alignments. It also adds first line with indent.
The second paragraph writes test with left alignment. It also adds first line with indent.
The third paragraph texts are italic with left alignment. The fourth paragraph texts are bold with left alignment.
The fifth paragraph texts are strike-through with left alignment. The sixth paragraph texts are undelined with left alignment.
Finally we create the output WordDocx.docx file in the root directory of the project. You can also save the file anywhere you want. This will create a word document using apache poi.
package com.roytuts.word.apache.poi;
import java.io.FileOutputStream;
import java.io.IOException;
import org.apache.poi.xwpf.usermodel.ParagraphAlignment;
import org.apache.poi.xwpf.usermodel.UnderlinePatterns;
import org.apache.poi.xwpf.usermodel.XWPFDocument;
import org.apache.poi.xwpf.usermodel.XWPFParagraph;
import org.apache.poi.xwpf.usermodel.XWPFRun;
public class WordDocx {
public static void main(String[] args) {
createWordDocument("WordDocx.docx");
}
public static void createWordDocument(final String outputFileName) {
// create a document
XWPFDocument doc = new XWPFDocument();
// create a paragraph with justify alignment
XWPFParagraph p1 = doc.createParagraph();
// first line indentation in the paragraph
p1.setFirstLineIndent(400);
// justify alignment
p1.setAlignment(ParagraphAlignment.DISTRIBUTE);
// wrap words
p1.setWordWrapped(true);
// insert page break after this paragraph
// p1.setPageBreak(true);
// XWPFRun object defines a region of text with a common set of
// properties
XWPFRun r1 = p1.createRun();
String t1 = "Sample Paragraph Post. This is a sample Paragraph post. Sample Paragraph text is being cut and pasted again and again. This is a sample Paragraph post. peru-duellmans-poison-dart-frog."
+ " Sample Paragraph Post. This is a sample Paragraph post. Sample Paragraph text is being cut and pasted again and again. This is a sample Paragraph post. peru-duellmans-poison-dart-frog.";
r1.setText(t1);
// create a paragraph with left alignment
XWPFParagraph p2 = doc.createParagraph();
// first line indentation in the paragraph
p2.setFirstLineIndent(400);
// left alignment
p2.setAlignment(ParagraphAlignment.LEFT);
// wrap words
p2.setWordWrapped(true);
// insert page break after this paragraph
// p2.setPageBreak(true);
// XWPFRun object defines a region of text with a common set of
// properties
XWPFRun r2 = p2.createRun();
String t2 = "Sample Paragraph Post. This is a sample Paragraph post. Sample Paragraph text is being cut and pasted again and again. This is a sample Paragraph post. peru-duellmans-poison-dart-frog."
+ " Sample Paragraph Post. This is a sample Paragraph post. Sample Paragraph text is being cut and pasted again and again. This is a sample Paragraph post. peru-duellmans-poison-dart-frog.";
r2.setText(t2);
// create a paragraph with ITALIC text
XWPFParagraph p3 = doc.createParagraph();
// left alignment
p3.setAlignment(ParagraphAlignment.LEFT);
// wrap words
p3.setWordWrapped(true);
// XWPFRun object defines a region of text with a common set of
// properties
XWPFRun r3 = p3.createRun();
String t3 = "Sample Paragraph Post. This is a sample Paragraph post. Sample Paragraph text is being cut and pasted again and again. This is a sample Paragraph post. peru-duellmans-poison-dart-frog.";
r3.setText(t3);
// make text italic
r3.setItalic(true);
// create a paragraph with BOLD text
XWPFParagraph p4 = doc.createParagraph();
// left alignment
p4.setAlignment(ParagraphAlignment.LEFT);
// wrap words
p4.setWordWrapped(true);
// XWPFRun object defines a region of text with a common set of
// properties
XWPFRun r4 = p4.createRun();
String t4 = "Sample Paragraph Post. This is a sample Paragraph post. Sample Paragraph text is being cut and pasted again and again. This is a sample Paragraph post. peru-duellmans-poison-dart-frog.";
r4.setText(t4);
// make text bold
r4.setBold(true);
// create a paragraph with Strike-Through text
XWPFParagraph p5 = doc.createParagraph();
// left alignment
p5.setAlignment(ParagraphAlignment.LEFT);
// wrap words
p5.setWordWrapped(true);
// XWPFRun object defines a region of text with a common set of
// properties
XWPFRun r5 = p5.createRun();
String t5 = "Sample Paragraph Post. This is a sample Paragraph post. Sample Paragraph text is being cut and pasted again and again. This is a sample Paragraph post. peru-duellmans-poison-dart-frog.";
r5.setText(t5);
// make StrikeThrough
r5.setStrikeThrough(true);
// create a paragraph with Underlined text
XWPFParagraph p6 = doc.createParagraph();
// left alignment
p6.setAlignment(ParagraphAlignment.LEFT);
// wrap words
p6.setWordWrapped(true);
// XWPFRun object defines a region of text with a common set of
// properties
XWPFRun r6 = p6.createRun();
String t6 = "Sample Paragraph Post. This is a sample Paragraph post. Sample Paragraph text is being cut and pasted again and again. This is a sample Paragraph post. peru-duellmans-poison-dart-frog.";
r6.setText(t6);
// make Underlined
r6.setUnderline(UnderlinePatterns.SINGLE);
// write to a docx file
FileOutputStream fo = null;
try {
// create .docx file
fo = new FileOutputStream(outputFileName);
// write to the .docx file
doc.write(fo);
} catch (IOException e) {
} finally {
if (fo != null) {
try {
fo.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (doc != null) {
try {
doc.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
Related Posts:
- add images to word document using apache poi
- create table in word document using apache poi
- create header and footer in word document using apache poi
Testing the Application
Run the above class to see the output in WordDocx.docx
file. The file is created under root directory of the project.
Now open the created word document you should see the expected output similar to below image.
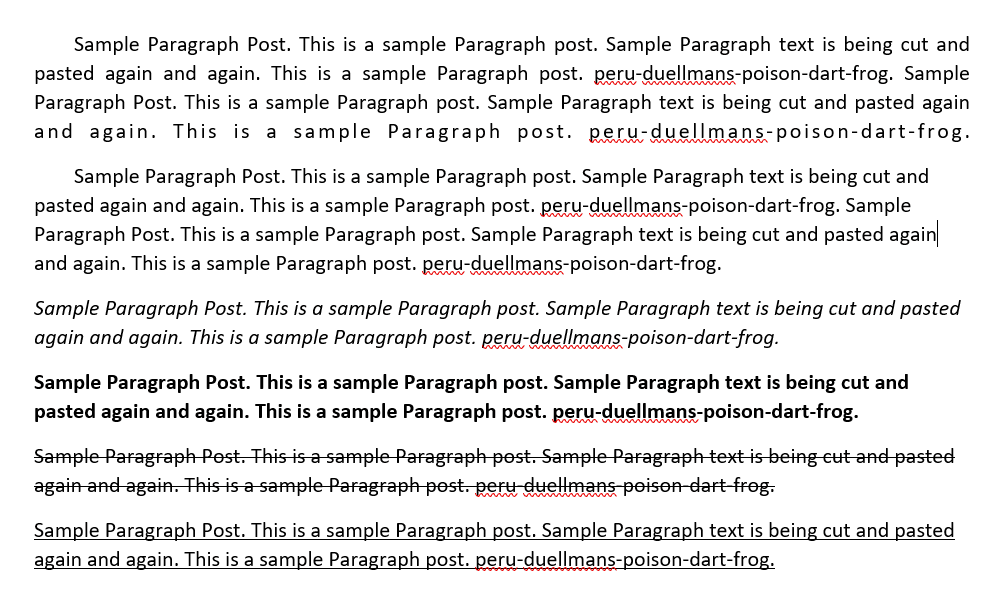
Hope you have got an idea how to create a word document using apache poi.
Source Code
Thanks for reading.