Introduction
Here I will show you ReactJs REST API GET Example. React is a declarative, efficient, and flexible JavaScript library for building user interface. In this tutorial I will show you how to fetch data from REST service using React JS framework. Here I am going to use sample and ready made available REST API https://jsonplaceholder.typicode.com/posts for testing.
You can also create and deploy your own service using PHP, Codeigniter or Spring, Jersey etc. and call using React JS framework. Here you will see only GET example.
Related Posts:
- https://roytuts.com/tic-tac-toe-game-using-react-js/
- ReactJS REST API POST Example
- ReactJS REST API PUT Example
- ReactJS REST API DELETE Example
Prerequisites
Knowledge of HTML and JavaScript https://jsonplaceholder.typicode.com/posts and Create React JS Application
Project Directory
Now I will see step by step how to implement the example using React JS or React framework. The project root directory is react-rest-get-api.
Now delete everything from the directory react-rest-get-api/src but do not delete src directory.
REST Controller
Create RestController.js
file under src directory with below content. Notice that you need to import the required module or component such as import React from ‘react’.
Here I have initialized table column headers in the constructor of the RestController
class. I have also declared users array to fill later with REST response data from https://jsonplaceholder.typicode.com/posts.
You can learn about componentDidMount()
at https://reactjs.org/docs/react-component.html.
import React from 'react';
class RestController extends React.Component {
constructor(props) {
super(props);
this.state = {users: []};
this.headers = [
{ key: 'userId', label: 'User ID' },
{ key: 'id', label: 'ID' },
{ key: 'title', label: 'Title' },
{ key: 'body', label: 'Body' }
];
}
componentDidMount() {
fetch('https://jsonplaceholder.typicode.com/posts')
.then(response => {
return response.json();
}).then(result => {
this.setState({
users:result
});
});
}
render() {
return (
<table>
<thead>
<tr>
{
this.headers.map(function(h) {
return (
<th key = {h.key}>{h.label}</th>
)
})
}
</tr>
</thead>
<tbody>
{
this.state.users.map(function(item, key) {
return (
<tr key = {key}>
<td>{item.userId}</td>
<td>{item.id}</td>
<td>{item.title}</td>
<td>{item.body}</td>
</tr>
)
})
}
</tbody>
</table>
)
}
}
export default RestController;
Export the RestController
at the end of the RestController
class so that you can use this class in other modules such as we have used it in below index.js file.
View File
Finally create index.js file under src directory to start up the application.
Here I have called <RestController/>
component and writing the output of the REST API’s GET response to the div id marked as root
.
Open the file react-rest-get-api/src/public/index.html, you will find a div with “root” id. Update the title in this file as “React – REST API GET Example”.
import React from 'react';
import ReactDOM from 'react-dom';
import RestController from './RestController'
ReactDOM.render(
<RestController />,
document.getElementById('root')
);
Testing the Application
Now execute the command npm start from command prompt on the project’s root directory and you will see the response data from https://jsonplaceholder.typicode.com/posts in browser at http://localhost:3000.
There are total 100 rows in the below table but here I am showing some of them in the image:
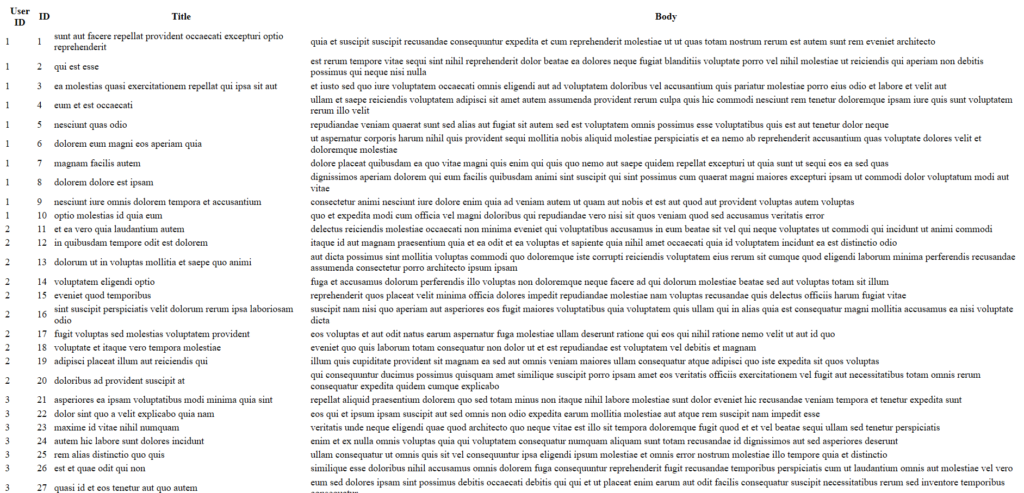
Source Code
Thanks for reading.
Great. I want to know that how to get single post using id as single post in new component? I have done this tutorial. Now I want to get single post using id.
Thanks In advance.