Introduction
In this tutorial I will show how to use SSL/TSL or https in JAX-WS based SOAP webservice. For using SSL with JAX-WS webservice you need one keystore file. I will use here Java tool to generate the keystore file. I also need to configure SSL in Tomcat server because I am going to deploy our application into Tomcat server.
Prerequisites
Please have a look at deploy jax-ws webservice into tomcat server before reading this tutorial.
Java at least 8, Gradle 6.5.1, JAX-WS API, Tomcat 9.0.24
Generating Keystore File
First generate the keystore file using the below command from the command prompt. C:\keystore the target location where the keystore file will be generated.
The file name is keystore.jks. You can also give a file name with .keystore.
C:\Java\jdk-12.0.2\bin\keytool -genkey -alias tomcat -keyalg RSA -keystore C:\keystore\keystore.jks
Make sure to change the JDK path in the above command with your system’s JDK path. Also if you want to change the keystore file location then you can change accordingly.
Next after executing the above command in the command line tool you will be prompted for below options. So enter the values as required.
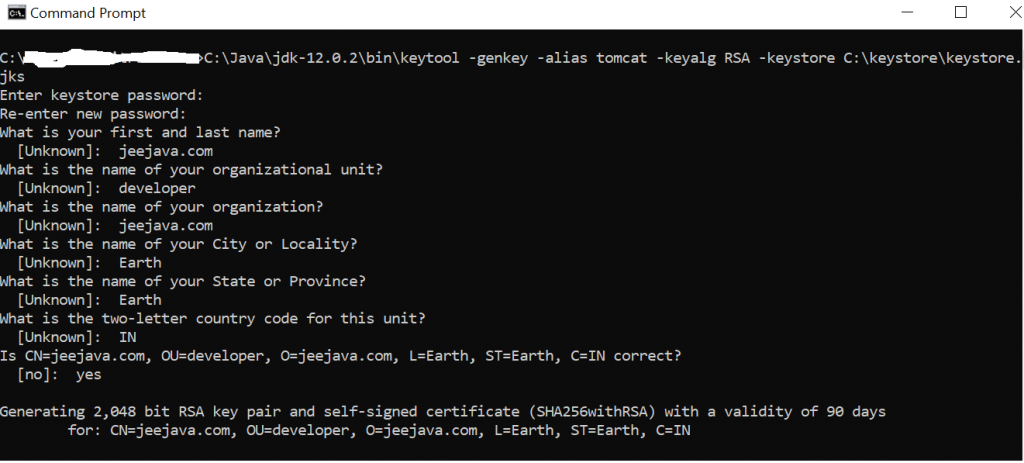
Text version of the above image:
Enter keystore password:
Re-enter new password:
What is your first and last name?
[Unknown]: roytuts.com
What is the name of your organizational unit?
[Unknown]: development
What is the name of your organization?
[Unknown]: roytuts.com
What is the name of your City or Locality?
[Unknown]: Earth
What is the name of your State or Province?
[Unknown]: Earth
What is the two-letter country code for this unit?
[Unknown]: IN
Is CN=roytuts.com, OU=development, O=roytuts.com, L=Earth, ST=Earth, C=IN correct?
[no]: yes
You may also like to read the similar example on:
SOAP over https with client certificate authentication
Configuring Tomcat to use SSL
Open <tomcat home directory>\conf\server.xml
file and search for <Connector port="8443" protocol="HTTP/1.1" SSLEnabled="true" .../>
If the above line is not commented out then comment the above line and add a new entry as shown below:
<Connector port="8443" protocol="org.apache.coyote.http11.Http11NioProtocol" SSLEnabled="true"
maxThreads="150" scheme="https" secure="true"
keystoreFile="file:///C:/keystore/keystore.jks"
keystorePass="password"
clientAuth="false" sslProtocol="TLS" />
For more information on SSL configuration you can have a look at the URL http://tomcat.apache.org/tomcat-7.0-doc/ssl-howto.html
Now start the Tomcat Server and verify whether SSL connection gets established using https://localhost:8443/ in the browser.
Accessing WSDL
Deploy the application into Tomcat server and try to access the WSDL file using SSL URL https://localhost:8443/jax-ws-webservice-tomcat/hello?wsdl from the browser.
You will get below output on the browser:
<definitions xmlns:wsu="http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-utility-1.0.xsd" xmlns:wsp="http://www.w3.org/ns/ws-policy" xmlns:wsp1_2="http://schemas.xmlsoap.org/ws/2004/09/policy" xmlns:wsam="http://www.w3.org/2007/05/addressing/metadata" xmlns:soap="http://schemas.xmlsoap.org/wsdl/soap/" xmlns:tns="http://impl.service.ws.jax.roytuts.com/" xmlns:xsd="http://www.w3.org/2001/XMLSchema" xmlns="http://schemas.xmlsoap.org/wsdl/" targetNamespace="http://impl.service.ws.jax.roytuts.com/" name="HelloImplService">
<import namespace="http://service.ws.jax.roytuts.com/" location="https://localhost:8443/jax-ws-webservice-tomcat/hello?wsdl=1"/>
<binding xmlns:ns1="http://service.ws.jax.roytuts.com/" name="HelloImplPortBinding" type="ns1:Hello">
<soap:binding transport="http://schemas.xmlsoap.org/soap/http" style="rpc"/>
<operation name="sayHello">
<soap:operation soapAction=""/>
<input>
<soap:body use="literal" namespace="http://service.ws.jax.roytuts.com/"/>
</input>
<output>
<soap:body use="literal" namespace="http://service.ws.jax.roytuts.com/"/>
</output>
</operation>
</binding>
<service name="HelloImplService">
<port name="HelloImplPort" binding="tns:HelloImplPortBinding">
<soap:address location="https://localhost:8443/jax-ws-webservice-tomcat/hello"/>
</port>
</service>
</definitions>
Testing the Service
Create a class with main method as shown below to test the JAX-WS based SOAP service for SSL.
package com.roytuts.jax.ws.service.consumer;
import java.net.URL;
import javax.xml.namespace.QName;
import javax.xml.ws.Service;
import com.roytuts.jax.ws.service.Hello;
public class HelloSSLClient {
public static void main(String[] args) throws Exception {
URL url = new URL("https://localhost:8443/jax-ws-webservice-tomcat/hello?wsdl");
QName qname = new QName("http://impl.service.ws.jax.roytuts.com/", "HelloImplService");
Service service = Service.create(url, qname);
Hello hello = service.getPort(Hello.class);
System.out.println(hello.sayHello("Soumitra"));
}
}
Executing the above class you will get below exceptions in localhost.
So click on the below exception links to resolve the issues.
- SunCertPathBuilderException: unable to find valid certification path to requested target
- java.security.cert.CertificateException: No name matching localhost found
Thanks for reading.